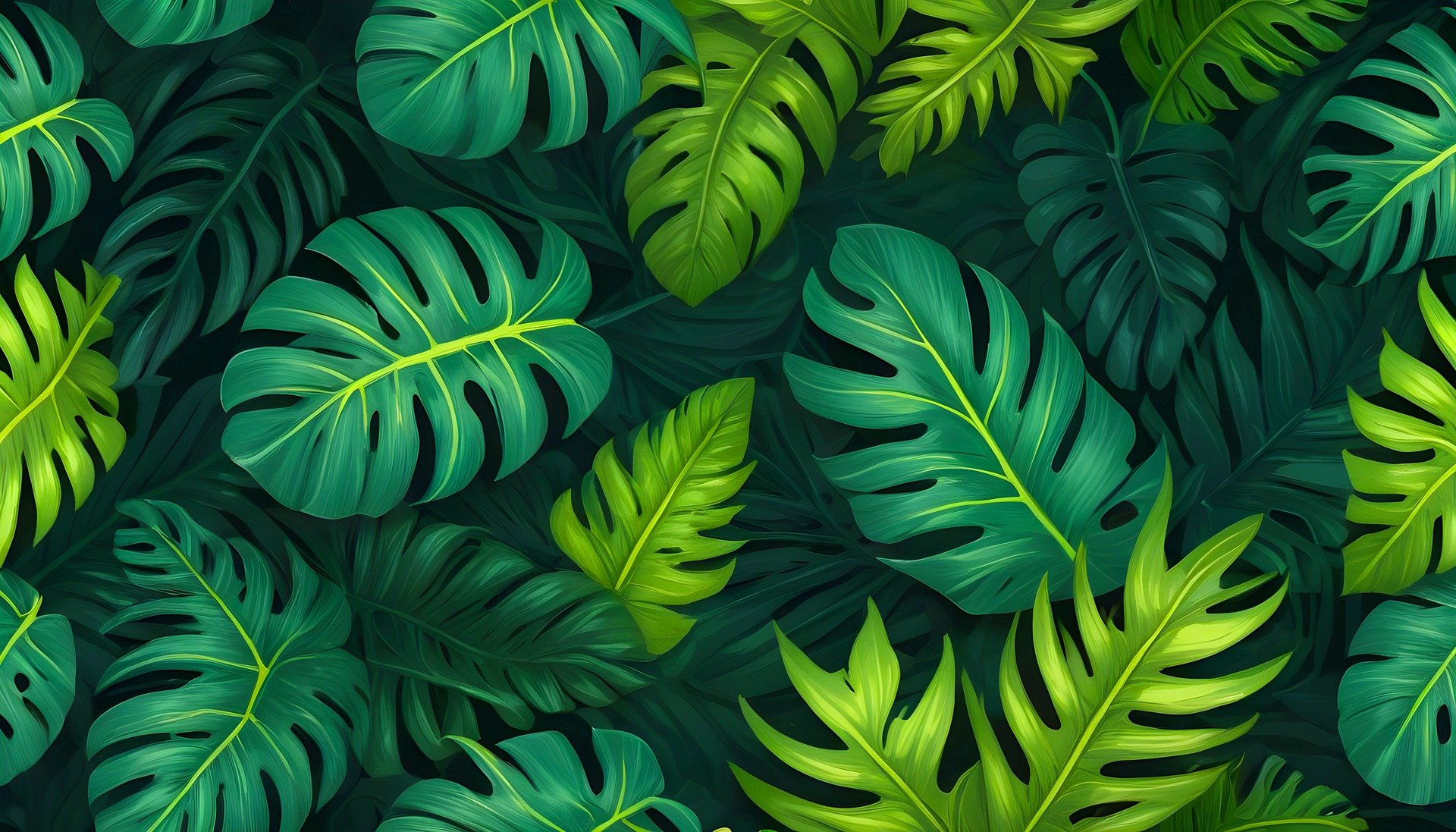
题目描述
199. 二叉树的右视图
给定一个二叉树的 根节点 root
,想象自己站在它的右侧,按照从顶部到底部的顺序,返回从右侧所能看到的节点值。
示例 1:
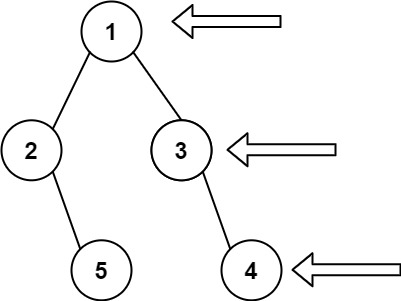
1 2
| 输入: [1,2,3,null,5,null,4] 输出: [1,3,4]
|
示例 2:
1 2
| 输入: [1,null,3] 输出: [1,3]
|
示例 3:
提示:
- 二叉树的节点个数的范围是
[0,100]
-100 <= Node.val <= 100
解题思路
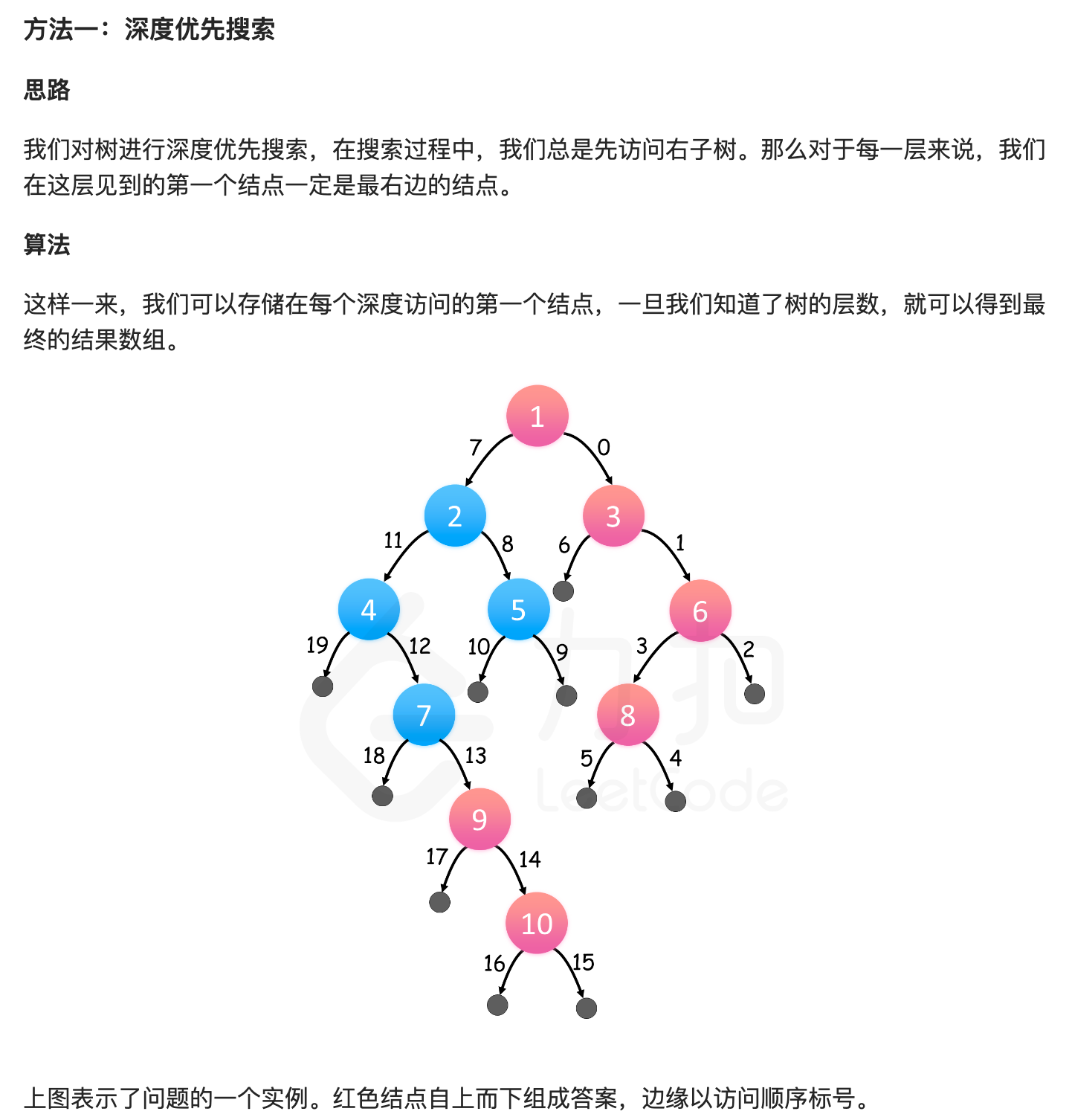
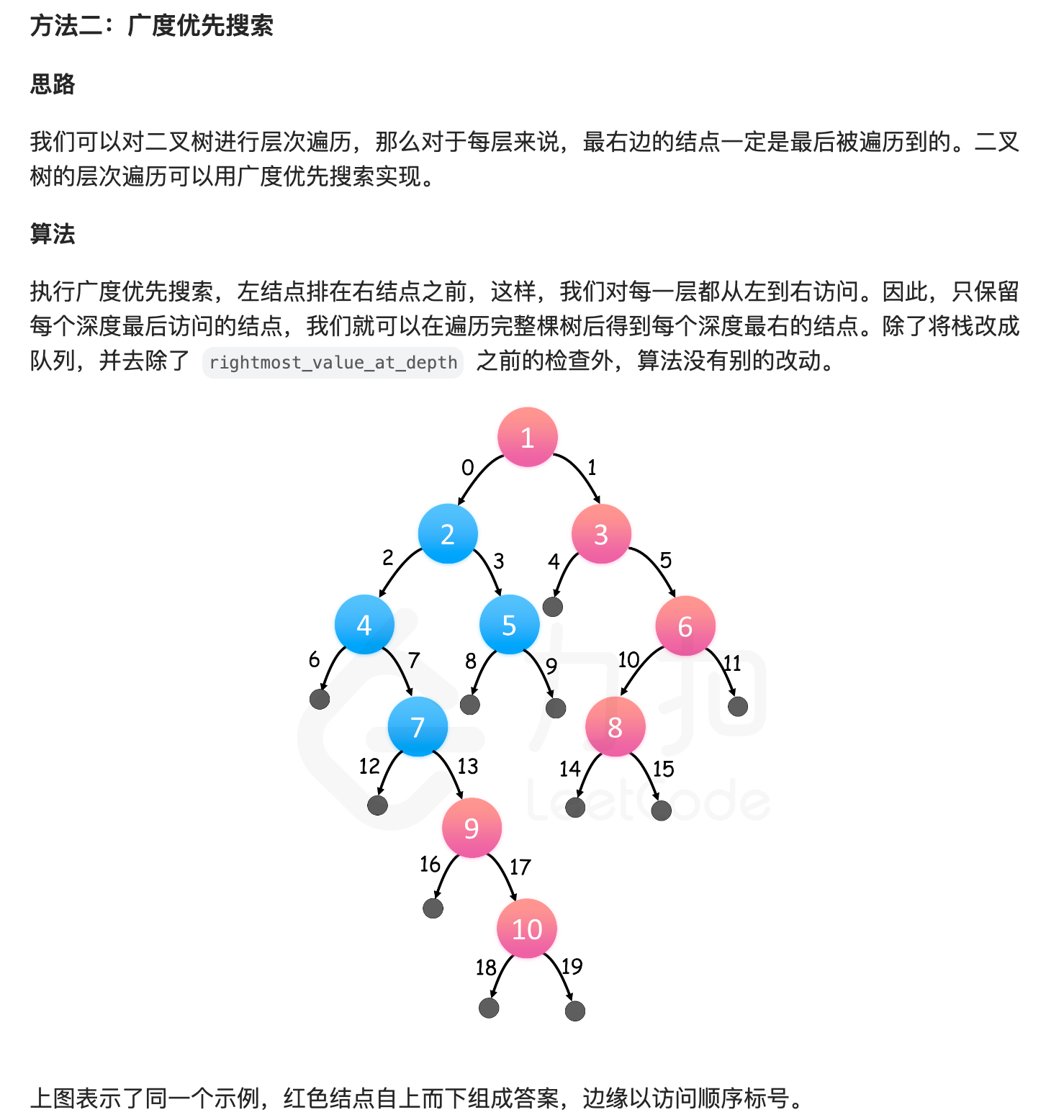
代码实现
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38
| public class Solution199Case1 { public List<Integer> rightSideView(TreeNode root) { Map<Integer, Integer> rightmostValueAtDepth = new HashMap<>(); int max_depth = -1;
Deque<TreeNode> nodeStack = new LinkedList<>(); Deque<Integer> depthStack = new LinkedList<>(); nodeStack.push(root); depthStack.push(0);
while (!nodeStack.isEmpty()) { TreeNode node = nodeStack.pop(); int depth = depthStack.pop();
if (node != null) { max_depth = Math.max(max_depth, depth);
if (!rightmostValueAtDepth.containsKey(depth)) { rightmostValueAtDepth.put(depth, node.val); }
nodeStack.push(node.left); nodeStack.push(node.right); depthStack.push(depth + 1); depthStack.push(depth + 1); } }
List<Integer> rightView = new ArrayList<Integer>(); for (int depth = 0; depth <= max_depth; depth++) { rightView.add(rightmostValueAtDepth.get(depth)); }
return rightView; } }
|