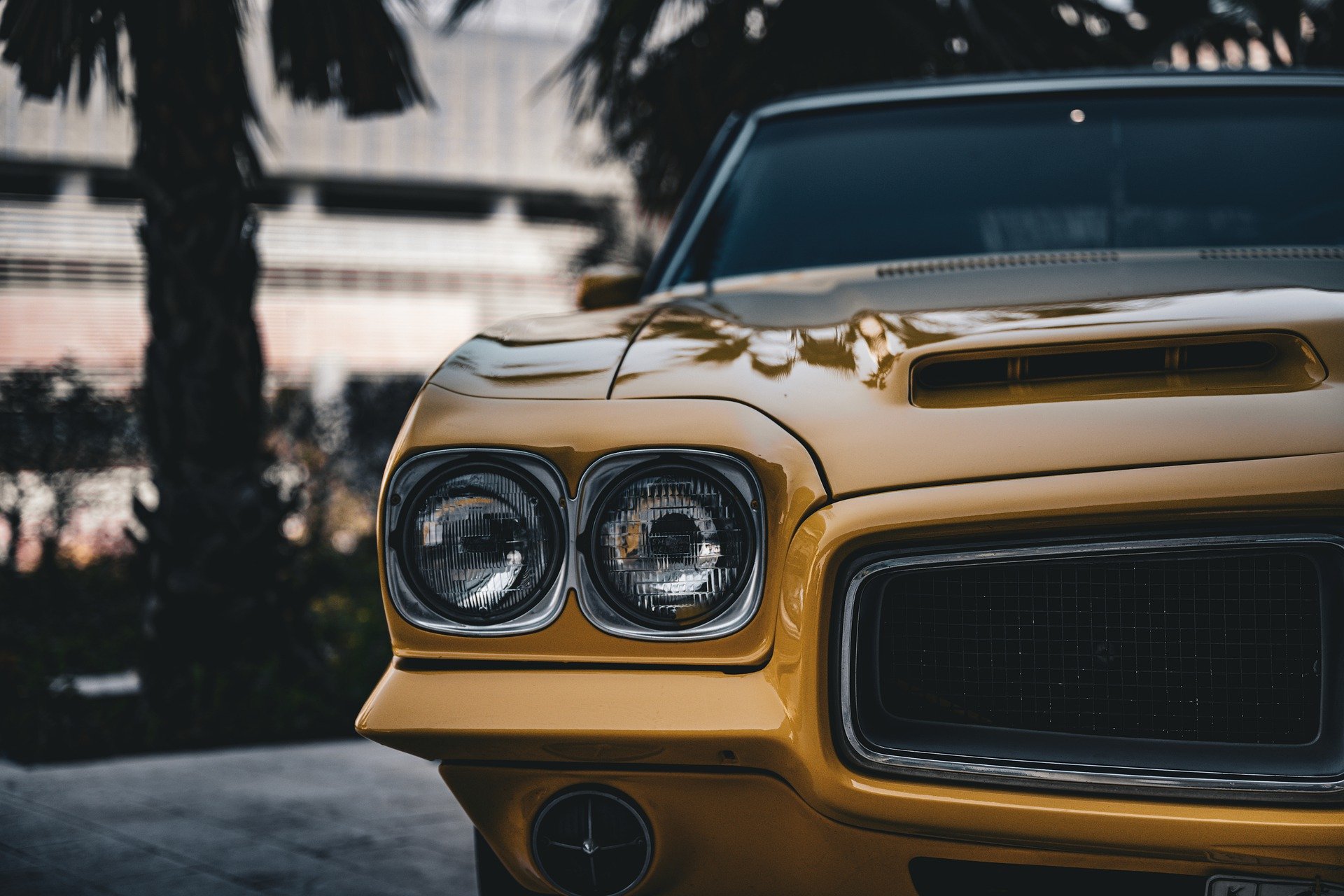
题目描述
238. 除自身以外数组的乘积
给你一个整数数组 nums
,返回 数组 answer
,其中 answer[i]
等于 nums
中除 nums[i]
之外其余各元素的乘积 。
题目数据 保证 数组 nums
之中任意元素的全部前缀元素和后缀的乘积都在 32 位 整数范围内。
请 不要使用除法,且在 O(*n*)
时间复杂度内完成此题。
示例 1:
1 2
| 输入: nums = [1,2,3,4] 输出: [24,12,8,6]
|
示例 2:
1 2
| 输入: nums = [-1,1,0,-3,3] 输出: [0,0,9,0,0]
|
提示:
2 <= nums.length <= 105
-30 <= nums[i] <= 30
- 保证 数组
nums
之中任意元素的全部前缀元素和后缀的乘积都在 32 位 整数范围内
进阶:你可以在 O(1)
的额外空间复杂度内完成这个题目吗?( 出于对空间复杂度分析的目的,输出数组 不被视为 额外空间。)
解题思路
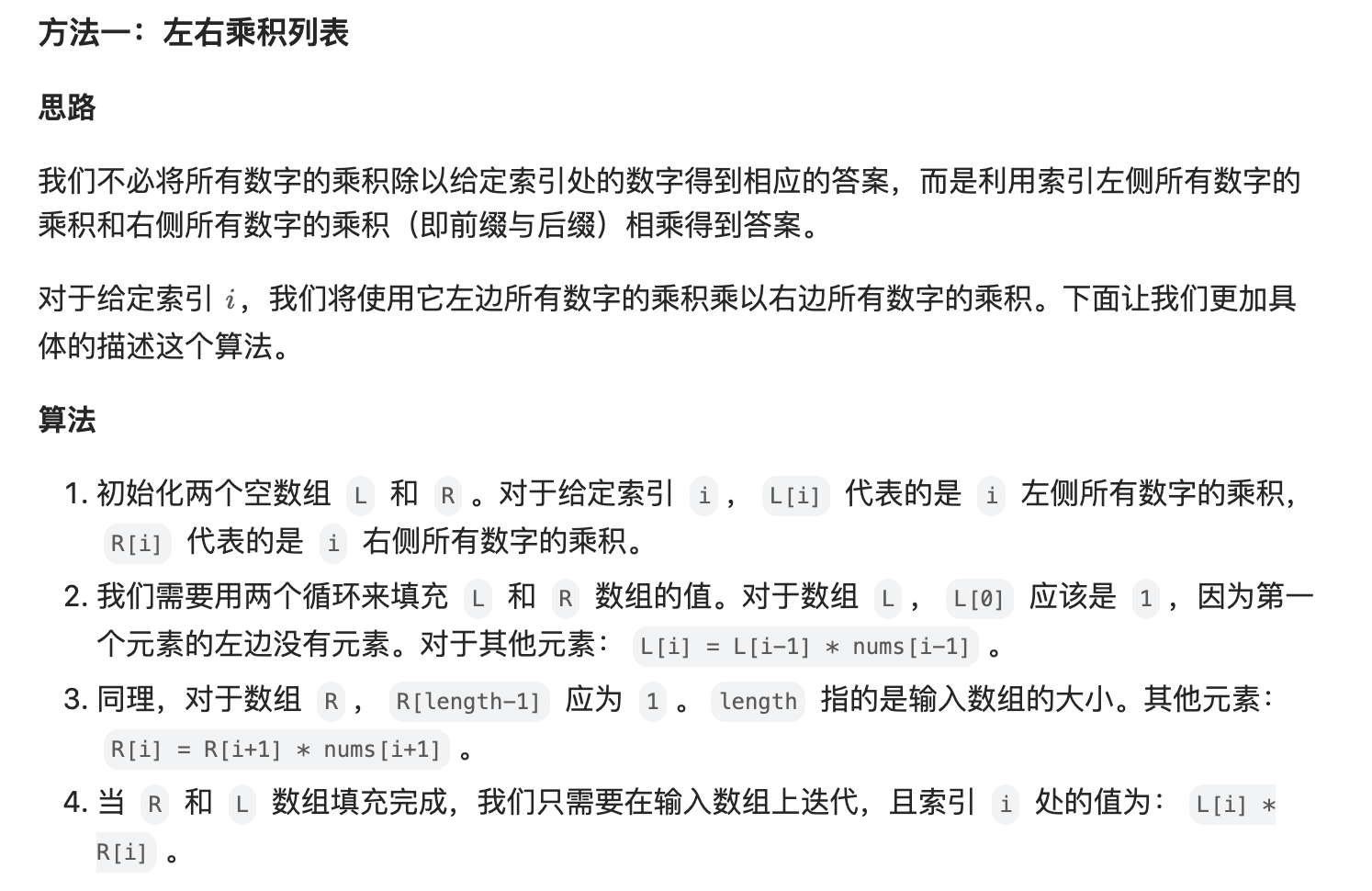
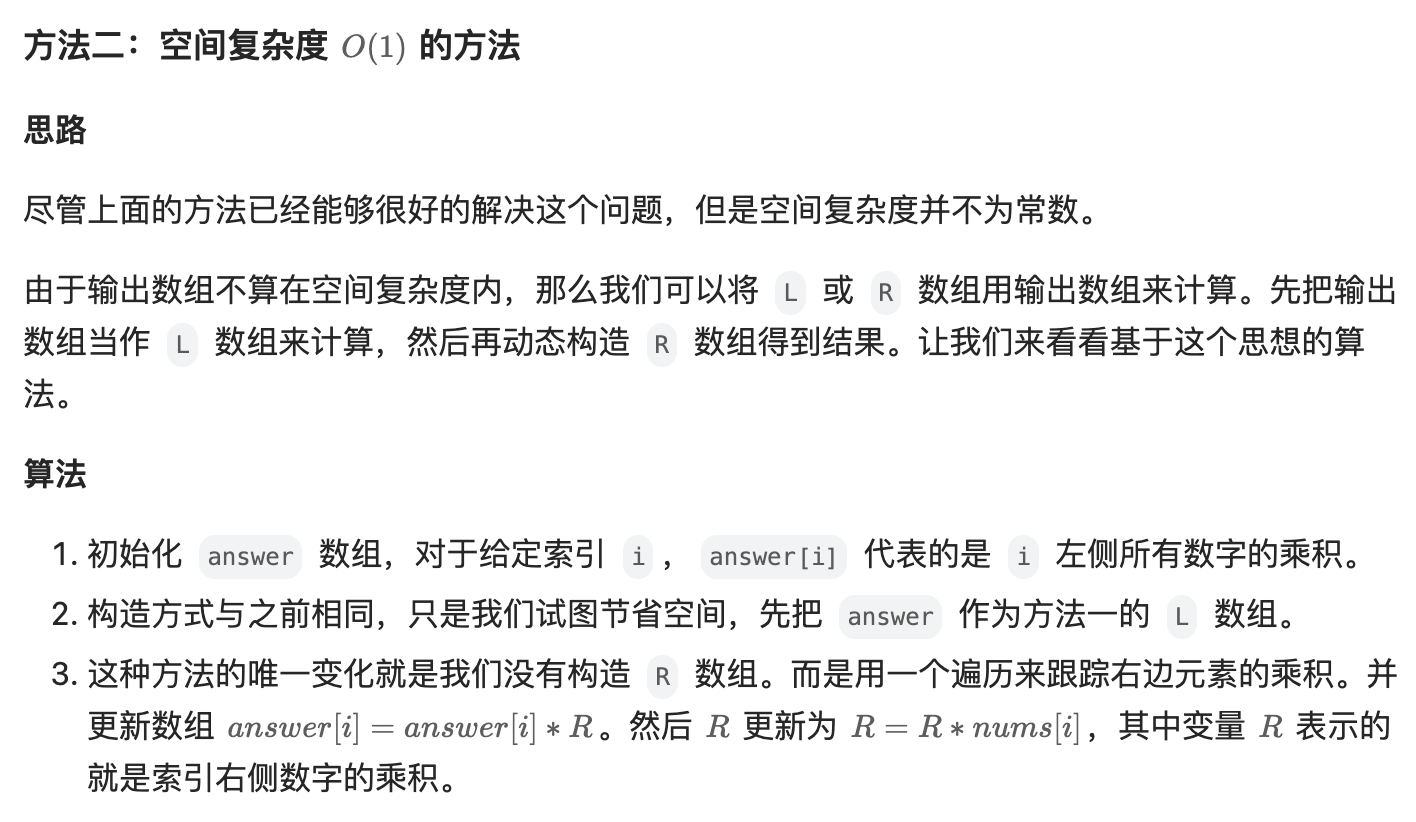
代码实现
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24
| public class Solution238Case1 { private static int[] productExceptSelf(int[] nums) { int[] L = new int[nums.length]; L[0] = 1; int[] R = new int[nums.length]; R[nums.length - 1] = 1; for (int i = 1; i < nums.length; i++) { L[i] = nums[i - 1] * L[i - 1]; } for (int i = nums.length - 2; i >= 0; i--) { R[i] = nums[i + 1] * R[i + 1]; } int[] result = new int[nums.length]; for (int i = 0; i < nums.length; i++) { result[i] = L[i] * R[i]; } return result; }
public static void main(String[] args) { int[] nums = {1, 2, 3, 4}; productExceptSelf(nums); } }
|
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19
| public class Solution238Case2 { private static int[] productExceptSelf(int[] nums) { int[] result = new int[nums.length]; result[0] = 1; for (int i = 1; i < nums.length; i++) { result[i] = nums[i - 1] * result[i - 1]; } int R = 1; for (int i = nums.length - 1; i >= 0; i--) { result[i] = result[i] * R; R *= nums[i]; } return result; }
public static void main(String[] args) {
} }
|