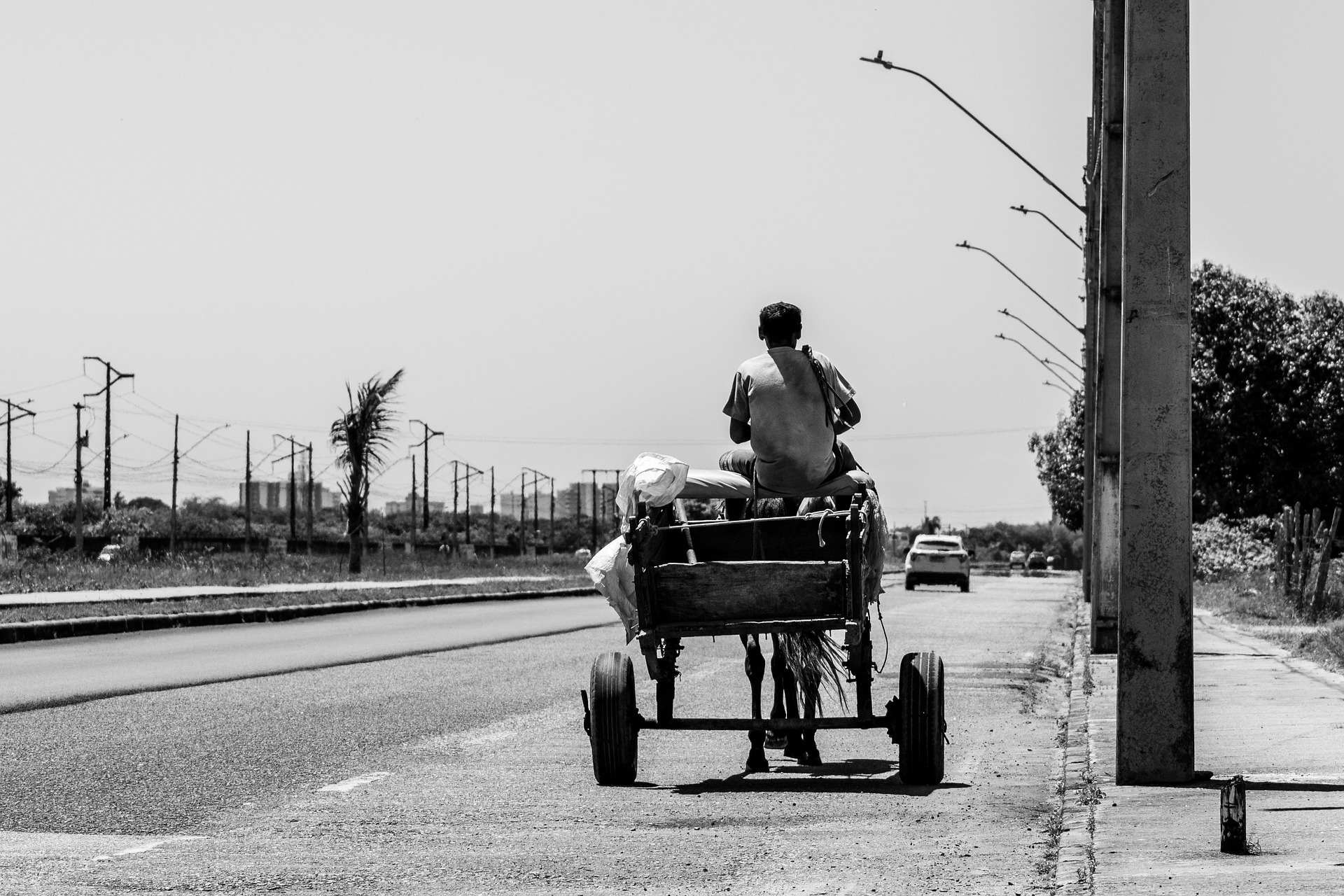
题目描述
49. 字母异位词分组
给你一个字符串数组,请你将 字母异位词 组合在一起。可以按任意顺序返回结果列表。
字母异位词 是由重新排列源单词的所有字母得到的一个新单词。
示例 1:
1 2
| 输入: strs = ["eat", "tea", "tan", "ate", "nat", "bat"] 输出: [["bat"],["nat","tan"],["ate","eat","tea"]]
|
示例 2:
1 2
| 输入: strs = [""] 输出: [[""]]
|
示例 3:
1 2
| 输入: strs = ["a"] 输出: [["a"]]
|
提示:
1 <= strs.length <= 104
0 <= strs[i].length <= 100
strs[i]
仅包含小写字母
解题思路
异位词:既两个字符串包含的字母相同
初始化一个 map,key 为排序后的异位词,value 为词组
- 将字符串排序
- 若 map 中已存在该字符串,将该字符串的原始字符串添加到词组中
- 若 map 中不存在该字符串,将该字符串的原始字符串添加到词组中
- 返回 map 的 value 集合
代码实现
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43
| public class Solution49 {
public static List<List<String>> groupAnagrams(String[] strs) { HashMap<String, List<String>> map = new HashMap<>(); for (String str : strs) { char[] chars = str.toCharArray(); Arrays.sort(chars); String s = new String(chars); List<String> list; if (map.containsKey(s)) { list = map.get(s); } else { list = new ArrayList<>(); map.put(s, list); } list.add(str); } return new ArrayList<>(map.values()); }
public static void main(String[] args) { String[] strs = {"eat", "tea", "tan", "ate", "nat", "bat"}; List<List<String>> list = groupAnagrams(strs); for (List<String> strings : list) { for (String string : strings) { System.err.println(string); } } } }
|