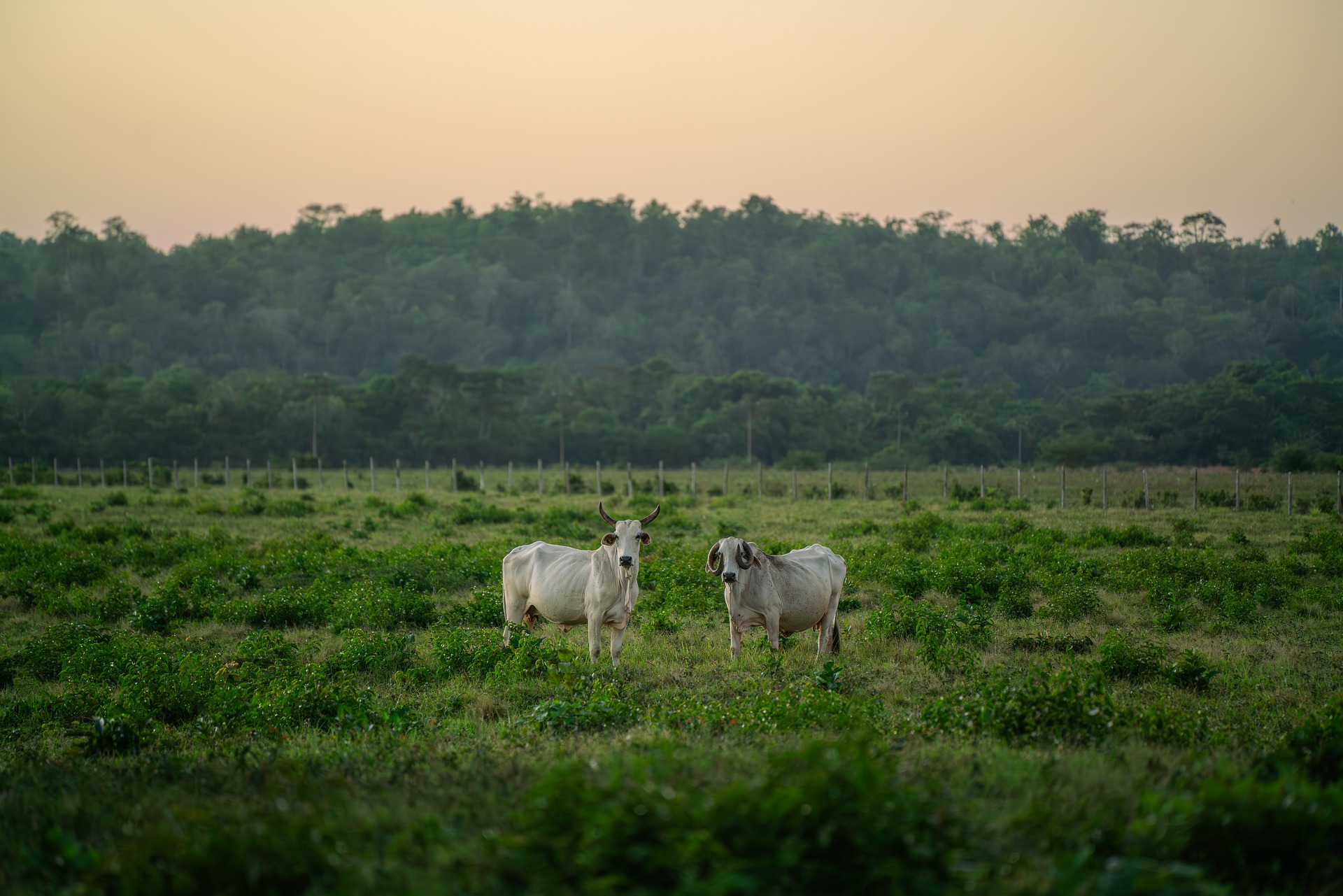
题目描述
面试题 17.04. 消失的数字
数组nums
包含从0
到n
的所有整数,但其中缺了一个。请编写代码找出那个缺失的整数。你有办法在O(n)时间内完成吗?
注意:本题相对书上原题稍作改动
示例 1:
示例 2:
1 2
| 输入:[9,6,4,2,3,5,7,0,1] 输出:8
|
解题思路
方法一:排序后与数组索引比较
- 将数组按照从小到大的顺序排序。
- 遍历排序后的数组,若当前元素值不等于索引,则索引值为缺失的整数。
- 若遍历结束仍未返回,则缺失的数为数组长度 + 1。
方法二:数学
利用等差数列求和公式:和=(首项+末项)×项数÷2。
- 利用求和公式求不缺失数字数组的和为
sum
。
- 对输入数组求和为
total
。
- 缺失的数为:sum - total。
代码实现
源码
方法一
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30
| public class Solution1704 { public static int missingNumber(int[] nums) { sort(nums); for (int i = 0; i < nums.length; i++) { if (nums[i] != i) { return i; } } return nums.length + 1; }
private static void sort(int[] arr) { for (int i = 0; i < arr.length; i++) { for (int j = i + 1; j < arr.length; j++) { if (arr[i] > arr[j]) { int temp = arr[i]; arr[i] = arr[j]; arr[j] = temp; } } } } }
|
方法二
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24
| public class Solution1704 {
public static int missingNumber(int[] nums) { int n = nums.length; int sum = (n * (n + 1)) / 2; int total = 0; for (int num : nums) { total += num; } return sum - total; }
public static void main(String[] args) { int[] nums = {9, 6, 4, 2, 3, 5, 7, 0, 1}; System.err.println(missingNumber(nums)); } }
|